Website performance is crucial for user experience and search engine rankings. WordPress sites with complex custom fields can experience slower load times if fields are loaded inefficiently. Lazy loading Advanced Custom Fields (ACF) with shortcodes offers an effective solution to improve page speed without sacrificing functionality. This guide explores how to implement lazy loading for ACF fields using shortcodes to optimize WordPress site performance.
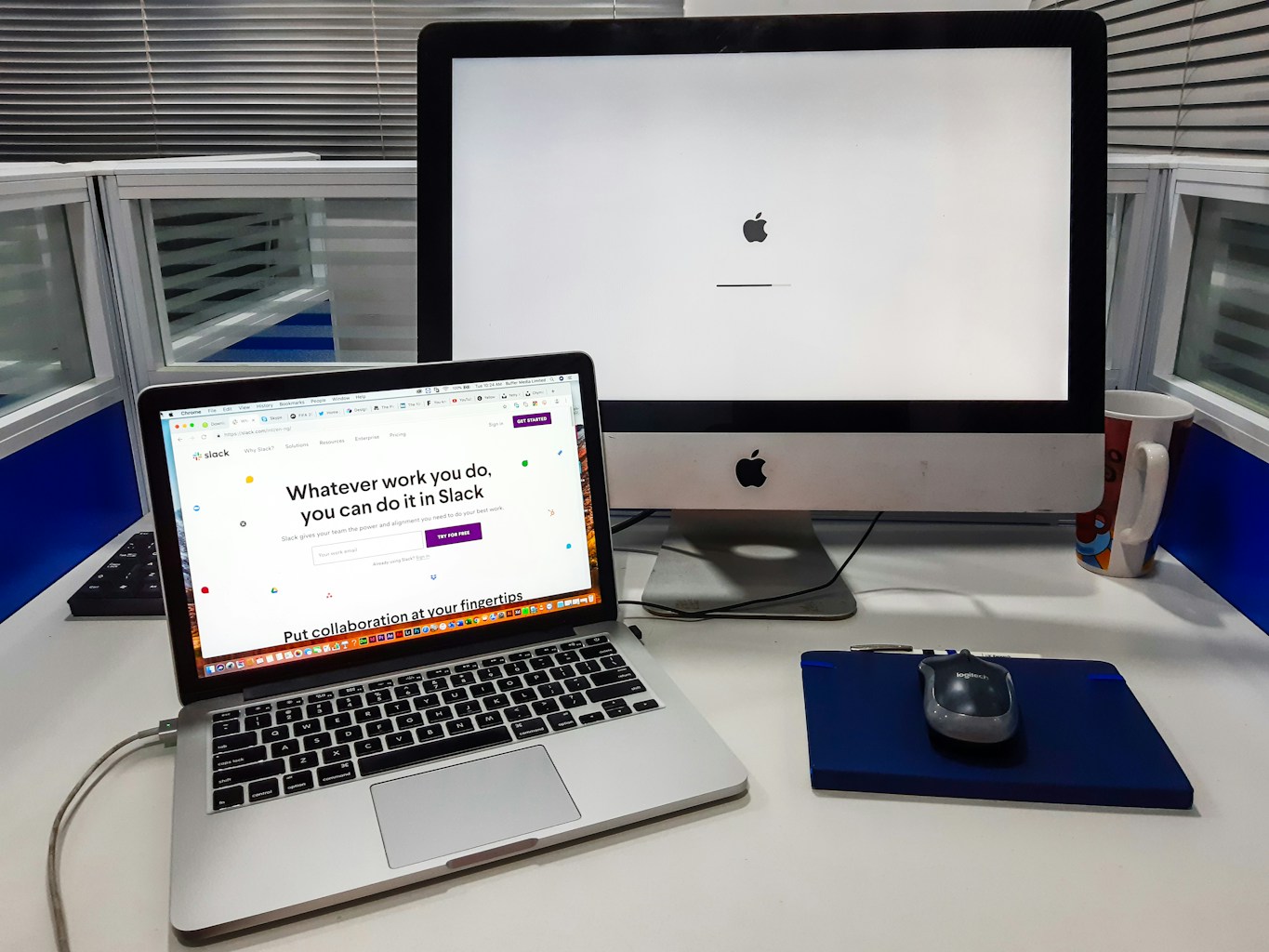
Why Lazy Loading Matters
Lazy loading defers the loading of non-essential elements until they are needed, reducing the initial page load time. For ACF fields, lazy loading ensures that only relevant fields are loaded dynamically, improving performance for content-heavy sites.
Benefits of Lazy Loading ACF Fields
- Improved Page Load Speed: Load only the required fields when they are needed.
- Reduced Server Load: Minimize database queries for custom fields.
- Enhanced User Experience: Deliver faster-loading pages without compromising functionality.
Learn more about lazy loading techniques in the WordPress developer documentation.
Setting Up ACF for Lazy Loading
Install ACF
Before implementing lazy loading, ensure ACF is installed and activated on your WordPress site.
- Navigate to Plugins > Add New in your WordPress dashboard.
- Search for “Advanced Custom Fields” and click Install Now.
- Click Activate once installed.
Create Custom Fields
- Go to Custom Fields in your dashboard.
- Click Add New to create a field group.
- Add fields relevant to your content, such as images, text, or links.
Example Fields for a Blog Post:
- Featured Quote (Text Field)
- Author Bio (Text Area)
- Related Articles (Relationship Field)
Assign the field group to specific post types, such as Posts or Pages, and publish it.
Understanding Shortcodes and Lazy Loading
What Are Shortcodes?
Shortcodes are simple text snippets that execute specific functionality in WordPress. For example, [acf_field]
could display custom field data dynamically within a post or page.
Why Use Shortcodes for Lazy Loading?
Shortcodes allow you to:
- Load ACF fields dynamically without modifying templates.
- Implement lazy loading by rendering content only when needed.
Implementing Lazy Loading for ACF Fields
Step 1: Create a Custom Shortcode
Add the following code to your theme’s functions.php
file or a custom plugin:
Basic Shortcode for ACF Fields:
function lazy_load_acf_field($atts) {
$atts = shortcode_atts(
array(
'field' => '',
'post_id' => get_the_ID(),
),
$atts
);
$field_value = get_field($atts['field'], $atts['post_id']);
if ($field_value) {
return esc_html($field_value);
}
return '<p>Loading...</p>'; // Placeholder text before content loads
}
add_shortcode('lazy_acf_field', 'lazy_load_acf_field');
Step 2: Use the Shortcode
In your WordPress editor, use the shortcode to display ACF fields:
[lazy_acf_field field="author_bio"]
This shortcode retrieves and displays the value of the Author Bio field.
Advanced Lazy Loading Techniques
Adding JavaScript for Asynchronous Loading
Enhance the shortcode by integrating JavaScript to load fields asynchronously after the page has loaded.
Step 1: Modify the Shortcode
Update the shortcode to output a placeholder with a unique ID:
function lazy_load_acf_field_async($atts) {
$atts = shortcode_atts(
array(
'field' => '',
'post_id' => get_the_ID(),
),
$atts
);
$unique_id = 'acf_field_' . $atts['field'] . '_' . $atts['post_id'];
return '<div id="' . esc_attr($unique_id) . '" class="lazy-acf-field" data-field="' . esc_attr($atts['field']) . '" data-post-id="' . esc_attr($atts['post_id']) . '">Loading...</div>';
}
add_shortcode('lazy_acf_field_async', 'lazy_load_acf_field_async');
Step 2: Add JavaScript
Enqueue a script to handle the asynchronous loading of custom fields:
function enqueue_lazy_load_script() {
wp_enqueue_script('lazy-load-acf', get_template_directory_uri() . '/js/lazy-load-acf.js', array('jquery'), null, true);
}
add_action('wp_enqueue_scripts', 'enqueue_lazy_load_script');
Create a lazy-load-acf.js
file in your theme’s js
folder:
jQuery(document).ready(function ($) {
$('.lazy-acf-field').each(function () {
var field = $(this).data('field');
var postId = $(this).data('post-id');
var container = $(this);
$.ajax({
url: '/wp-admin/admin-ajax.php',
type: 'POST',
data: {
action: 'get_lazy_acf_field',
field: field,
post_id: postId,
},
success: function (response) {
container.html(response);
},
});
});
});
Step 3: Handle AJAX Requests
Add an AJAX handler to fetch the field data:
function get_lazy_acf_field() {
$field = $_POST['field'] ?? '';
$post_id = $_POST['post_id'] ?? 0;
if ($field && $post_id) {
$field_value = get_field($field, $post_id);
echo esc_html($field_value);
}
wp_die();
}
add_action('wp_ajax_get_lazy_acf_field', 'get_lazy_acf_field');
add_action('wp_ajax_nopriv_get_lazy_acf_field', 'get_lazy_acf_field');
Optimizing Lazy Loading
Use Caching for Frequently Accessed Fields
Combine lazy loading with caching to further reduce server load and database queries.
Example Code for Caching Fields:
function lazy_load_cached_acf_field($atts) {
$atts = shortcode_atts(
array(
'field' => '',
'post_id' => get_the_ID(),
),
$atts
);
$cache_key = 'acf_field_' . $atts['field'] . '_' . $atts['post_id'];
$cached_value = get_transient($cache_key);
if (!$cached_value) {
$cached_value = get_field($atts['field'], $atts['post_id']);
set_transient($cache_key, $cached_value, 12 * HOUR_IN_SECONDS);
}
return $cached_value ? esc_html($cached_value) : '';
}
add_shortcode('lazy_cached_acf_field', 'lazy_load_cached_acf_field');
Optimize Field Queries
Minimize the number of fields queried by using specific field names or keys instead of loading entire field groups.
Real-World Applications
Portfolio Websites
Lazy load project details or media galleries to improve performance on portfolio pages.
Example Usage:
[lazy_acf_field_async field="project_description"]
E-Commerce Stores
Use lazy loading for product specifications, reviews, or FAQs to improve load times on product pages.
Blogs
Enhance user experience by lazily loading related posts, author bios, or custom metadata on blog pages.
Conclusion
Lazy loading ACF fields with shortcodes is a powerful strategy for improving WordPress site performance. By dynamically loading only the necessary custom field data and combining this approach with caching and asynchronous loading, you can significantly enhance page load times and user experience.
For more information on optimizing WordPress performance, visit the ACF documentation and explore additional techniques in the WordPress developer guides. Start implementing lazy loading today to deliver faster, more efficient websites!