Custom fields are a powerful feature in WordPress, enabling developers to add, display, and manage additional metadata for posts, pages, or custom post types. However, issues with custom fields can arise due to misconfigurations, theme or plugin conflicts, or even database errors. This guide provides developers with actionable steps and tools to debug custom field issues efficiently, ensuring smooth WordPress development.
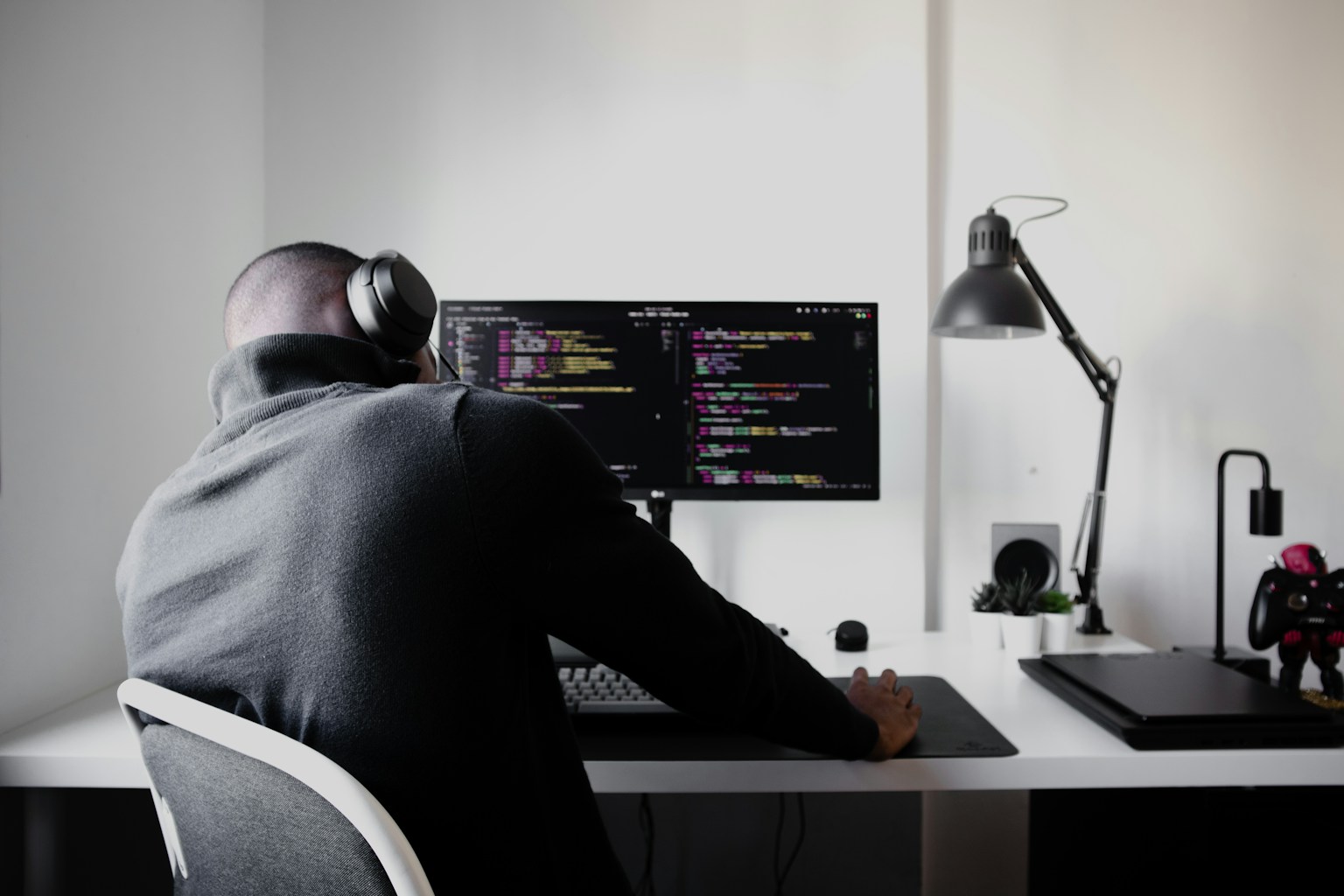
Common Custom Field Issues
Before diving into debugging, it’s essential to understand the common problems developers encounter with custom fields.
Missing Custom Field Data
- Symptoms: Custom fields don’t appear in the WordPress admin panel or on the frontend.
- Causes: Incorrect field setup, theme compatibility issues, or database errors.
Incorrect Field Output
- Symptoms: Custom fields display unexpected or incorrect data.
- Causes: Misconfigured field names or faulty PHP templates.
Performance Bottlenecks
- Symptoms: Pages with custom fields load slowly.
- Causes: Inefficient queries, excessive use of repeater fields, or lack of caching.
Learn more about managing custom fields in the official WordPress documentation.
Tools for Debugging Custom Fields
Enable WordPress Debug Mode
WordPress debug mode is an essential tool for identifying issues in your custom field setup.
Steps to Enable Debug Mode
- Open your
wp-config.php
file. - Add or update the following lines:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
define('WP_DEBUG_DISPLAY', false); // Optional: Hides errors on the frontend
- Check the debug log in the
wp-content/debug.log
file for error messages.
Use Query Monitor
The Query Monitor plugin provides insights into database queries, PHP errors, and performance bottlenecks related to custom fields.
How to Use Query Monitor:
- Install and activate the Query Monitor plugin.
- Load the page with custom fields to identify slow queries or errors.
- Review query data under the “Queries by Component” section.
Step-by-Step Debugging Process
Step 1: Verify Field Configuration
Start by checking the custom field settings in your ACF or custom plugin interface.
Check Field Names and Keys
- Ensure field names match the keys used in your templates.
- For ACF users, verify field group settings, including location rules and conditional logic.
Example: A missing field could result from using get_field('project_title')
when the field key is actually project_name
.
Step 2: Test Field Display in Templates
Use PHP functions like get_field()
or get_post_meta()
to test whether custom fields return data.
Example Code for Testing Field Output
<?php
$field_value = get_field('custom_field_name');
if ($field_value) {
echo '<p>' . esc_html($field_value) . '</p>';
} else {
echo '<p>Custom field is empty or not set.</p>';
}
?>
Step 3: Temporarily Switch to a Default Theme
Theme conflicts can prevent custom fields from displaying correctly. Switch to a default WordPress theme (like Twenty Twenty-Three) to rule out theme-related issues.
How to Switch Themes
- Go to Appearance > Themes in your WordPress dashboard.
- Activate a default theme.
- Test your custom fields again.
Debugging Specific Issues
Missing Custom Fields in Admin
Possible Causes
- The field group is not assigned to the correct location.
- User permissions restrict access to specific fields.
Solution
- Revisit your field group settings and check the Location Rules.
- Verify user roles and permissions using a plugin like Members.
Incorrect Data Display on Frontend
Possible Causes
- Template files reference the wrong field names.
- Data is not saved correctly in the database.
Solution
- Double-check the field names in your code against the ACF or custom field setup.
- Use the
get_post_meta()
function to inspect raw field data.
Example Code:
<?php
$raw_data = get_post_meta(get_the_ID(), 'custom_field_name', true);
var_dump($raw_data); // Debug raw field data
?>
Slow Page Loads with Custom Fields
Possible Causes
- Excessive database queries for complex field setups (e.g., repeater fields).
- Lack of caching for frequently accessed fields.
Solution
- Use the Transients API to cache field data.
Example Code for Caching Fields:
<?php
$cached_field = get_transient('field_cache');
if (!$cached_field) {
$cached_field = get_field('custom_field_name');
set_transient('field_cache', $cached_field, 12 * HOUR_IN_SECONDS);
}
echo $cached_field;
?>
- Optimize queries by limiting the number of fields retrieved.
Testing Advanced Custom Fields
Test Conditional Logic
If fields are not appearing due to conditional logic, review your rules in the field group editor.
Example Use Case: Display a “Sale Price” field only when a “Discount” checkbox is selected.
- Check if the logic is set correctly in ACF.
- Test by toggling the checkbox and observing field behavior.
Test Relationship and Repeater Fields
Complex fields like relationships or repeaters may not behave as expected. Use loop debugging to identify issues.
Example Code for Repeater Fields
<?php
if (have_rows('repeater_field')) {
while (have_rows('repeater_field')) {
the_row();
echo '<p>' . esc_html(get_sub_field('sub_field_name')) . '</p>';
}
} else {
echo '<p>No data found in repeater field.</p>';
}
?>
Optimizing Custom Field Performance
Minimize Unnecessary Queries
Avoid querying the same field multiple times on a single page. Store field values in variables and reuse them as needed.
Example:
<?php
$project_title = get_field('project_title');
if ($project_title) {
echo '<h1>' . esc_html($project_title) . '</h1>';
// Use $project_title again without re-querying
}
?>
Use Object Caching
Object caching can significantly reduce database load for custom field-heavy pages.
Real-World Scenarios
Portfolio Websites
Issue: Missing project details on portfolio pages.
Solution: Verified field keys and corrected template files to reference the correct custom fields.
E-Commerce Stores
Issue: Slow product pages with extensive repeater fields.
Solution: Optimized database queries and implemented caching for product specifications.
Membership Platforms
Issue: User profile fields not displaying for certain roles.
Solution: Updated field group permissions and tested role-specific logic.
Conclusion
Debugging custom field issues in WordPress doesn’t have to be a daunting task. By using tools like WordPress debug mode, Query Monitor, and caching, developers can efficiently identify and resolve problems.
Whether you’re working on portfolio sites, e-commerce stores, or membership platforms, following the steps in this guide ensures that your custom fields function as intended.
For additional resources, explore the ACF documentation and WordPress developer guides. Start debugging your custom fields today to deliver seamless and dynamic WordPress experiences!