ACF relationship fields provide WordPress developers with an efficient way to connect and manage complex data models. Advanced Custom Fields (ACF) is a cornerstone tool for creating dynamic and structured content, and relationship fields enhance its functionality by enabling seamless connections between posts, pages, or custom post types. This guide dives into the full potential of ACF relationship fields, offering actionable tips and best practices for their use in WordPress development.
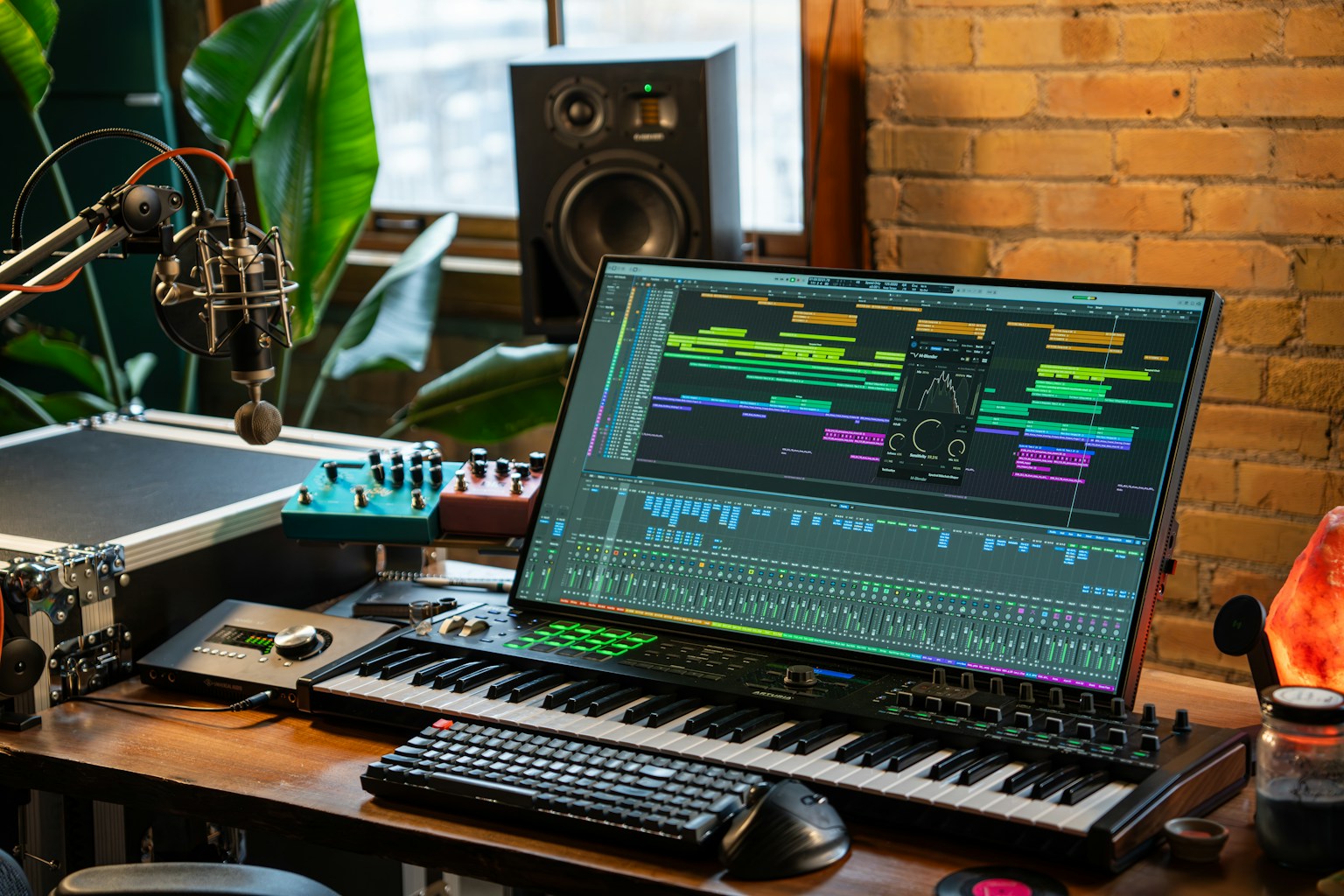
What Are ACF Relationship Fields?
ACF relationship fields allow you to link content across posts, pages, or custom post types in WordPress. These fields create dynamic connections between related content, providing an intuitive way to manage relationships within the WordPress admin panel.
For example:
- A real estate website can connect properties to agents.
- A recipe blog can link ingredients to individual recipes.
- An e-commerce store can associate products with collections or categories.
Learn more about the relationship field type in the ACF documentation.
Why Use Relationship Fields for Complex Data Models?
Relationship fields are essential for managing structured and interconnected content in WordPress. Benefits include:
- Improved Data Organization: Keep related content grouped and accessible.
- Dynamic Content Presentation: Easily display related posts, products, or custom data on the frontend.
- Streamlined Admin Experience: Enable editors to manage content relationships without writing code.
This functionality is invaluable for developers working on projects that require structured, relational data.
Setting Up ACF Relationship Fields
Step 1: Create the Relationship Field
- Navigate to Custom Fields in your WordPress admin panel.
- Click Add New to create a new field group.
- Add a new field and set its type to Relationship.
- Configure the field’s settings:
- Specify post types to relate (e.g., “Products” and “Categories”).
- Set filters for narrowing down choices in the admin panel.
- Enable return formats for more control over field output.
Step 2: Assign the Field Group
Assign the field group to specific post types or templates. For example, you might assign a “Related Products” field to the Product post type.
Refer to the field group creation guide for more detailed instructions.
Displaying Relationship Fields on the Frontend
Basic Example
To display the selected related posts or items on your site, use the get_field()
function.
<?php
$related_posts = get_field('related_posts');
if ($related_posts) {
echo '<ul>';
foreach ($related_posts as $post) {
setup_postdata($post);
echo '<li><a href="' . get_permalink() . '">' . get_the_title() . '</a></li>';
}
wp_reset_postdata();
echo '</ul>';
}
?>
In this example, the field related_posts
fetches the related content and displays it as a list of links.
Using Custom Queries
For more control, use WP_Query
to fetch and display relationship data.
<?php
$related_posts = get_field('related_posts');
if ($related_posts) {
$args = array(
'post_type' => 'post',
'post__in' => $related_posts,
'orderby' => 'post__in'
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
echo '<h2><a href="' . get_permalink() . '">' . get_the_title() . '</a></h2>';
}
wp_reset_postdata();
}
}
?>
This approach gives you more flexibility, such as customizing the query order or filtering results further.
Advanced Use Cases for Relationship Fields
Connecting Custom Post Types
Relationship fields are perfect for connecting custom post types, such as linking authors to books or courses to instructors.
- Use plugins like Custom Post Type UI to create post types.
- Set up ACF relationship fields to establish the connections.
- Display the relationships dynamically in single post templates or archives.
Explore CPT UI to manage custom post types easily.
Filtering Related Content
ACF relationship fields can be paired with filters to refine the data displayed on the frontend. For example, use query parameters to show related posts from a specific category or taxonomy.
Dynamic Widgets or Sidebars
Use relationship fields to populate dynamic widgets or sidebars with related content. For instance, show “Related Products” in a WooCommerce store or “Related Articles” on a blog.
Learn more about WooCommerce and ACF integration.
Performance Optimization
Limit the Number of Related Items
To prevent performance bottlenecks, limit the number of related items displayed using field settings or query parameters.
Cache Relationship Field Queries
For frequently accessed relationship data, implement caching using the WordPress Transients API.
<?php
$related_posts = get_transient('related_posts_' . get_the_ID());
if (!$related_posts) {
$related_posts = get_field('related_posts');
set_transient('related_posts_' . get_the_ID(), $related_posts, 12 * HOUR_IN_SECONDS);
}
// Use $related_posts as needed
?>
This reduces database load and speeds up page rendering.
Optimize Database Queries
When working with large datasets, use optimized queries and indexes to improve performance. Tools like Query Monitor can help identify slow queries.
Learn more about query optimization.
Testing and Debugging
Test for Empty Fields
Always check whether a relationship field contains data before displaying it.
<?php if (!empty($related_posts)) : ?>
<!-- Display related posts -->
<?php endif; ?>
Debug with Query Monitor
Use the Query Monitor plugin to debug relationship field queries and ensure they perform efficiently.
Explore Query Monitor for in-depth performance analysis.
Real-World Examples
Real Estate Website
A real estate site can use relationship fields to connect properties with agents. This setup allows visitors to view agent details on property pages and vice versa.
Recipe Blog
Relationship fields can link recipes with ingredients, creating a structured way to display interconnected data. For example, each ingredient can list all the recipes it’s used in.
E-Commerce Store
Online stores can associate products with categories, collections, or upsells using relationship fields. This enhances navigation and encourages cross-selling.
Conclusion
Mastering ACF relationship fields is essential for building complex data models in WordPress. Whether you’re managing interconnected custom post types, creating dynamic widgets, or optimizing performance, relationship fields offer unmatched flexibility and functionality.
By combining ACF relationship fields with best practices for querying and performance optimization, you can deliver highly functional, user-friendly WordPress sites. For more resources, visit the official ACF documentation.
Start exploring the potential of ACF relationship fields today and elevate your WordPress development projects to the next level!